How Next.js Websites Store Data: A Comprehensive Guide

Next.js is a powerful React framework that has gained immense popularity for its ability to create high-performance web applications. However, a common question arises among developers: How do Next.js websites store and manage data effectively? In this interactive guide, we’ll explore everything you need to know about data storage in Next.js applications, covering every aspect of the topic in an easy-to-understand format.
1. Introduction to Data Handling in Next.js
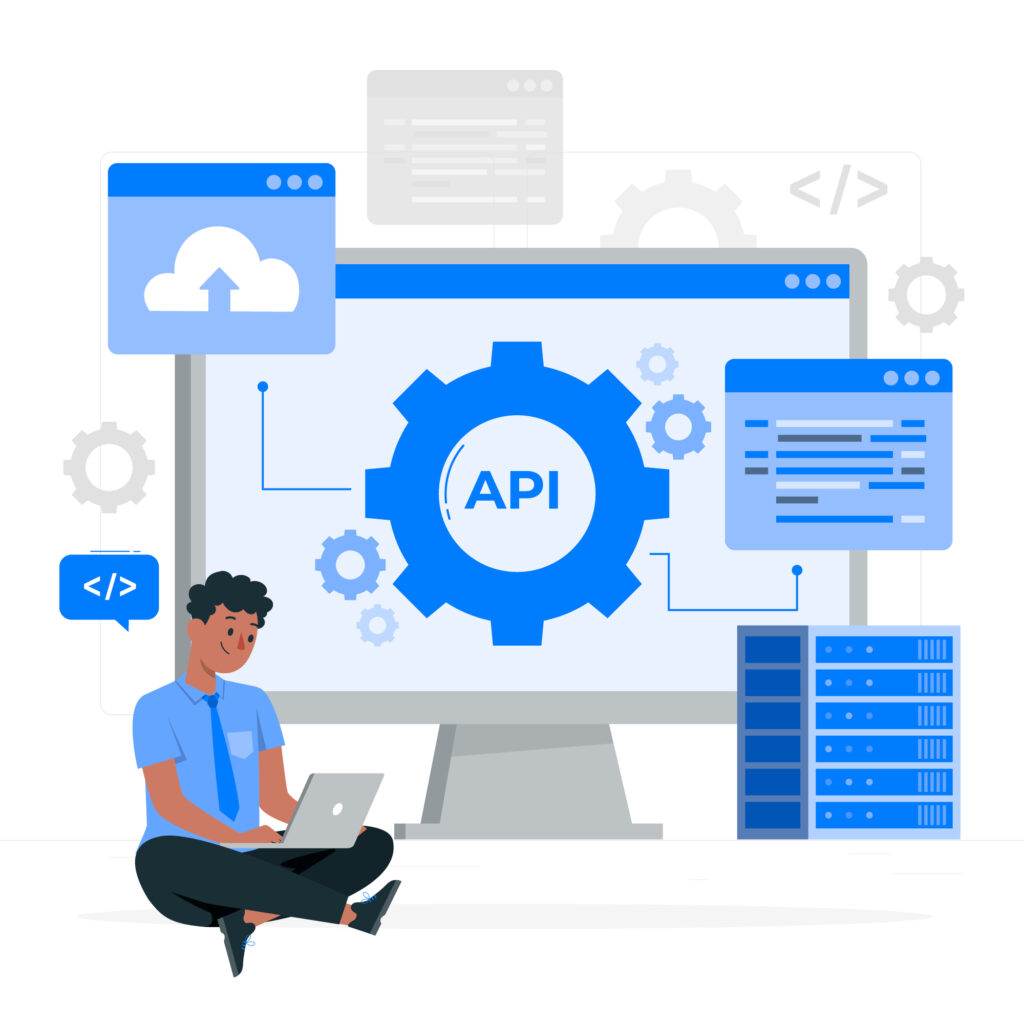
Next.js simplifies web development by combining server-side rendering (SSR), static site generation (SSG), and dynamic routing. However, choosing the right data storage and fetching mechanism can make or break your application’s performance.
In this guide, you’ll learn:
- How to fetch data in Next.js using different methods.
- The pros and cons of various storage solutions.
- Practical tips to enhance security and performance.
2. Data Fetching Methods in Next.js
Next.js offers flexible data fetching methods to suit various use cases. Let’s break them down:
getStaticProps
Fetch data at build time to generate static pages. Ideal for content that doesn’t change frequently.
export async function getStaticProps() {
const res = await fetch('https://api.example.com/data');
const data = await res.json();
return {
props: { data },
};
}
- Use Cases: Blogs, documentation sites.
- Benefits: Fast performance, great for SEO.
getServerSideProps
Fetch data on every request, ensuring up-to-date content.
export async function getServerSideProps(context) {
const res = await fetch(`https://api.example.com/data?id=${context.params.id}`);
const data = await res.json();
return {
props: { data },
};
}
- Use Cases: Dashboards, dynamic data-driven pages.
- Benefits: Real-time updates.
API Routes
Define backend endpoints within your Next.js app using the /app/api
directory.
// pages/api/hello.js
export default function handler(req, res) {
res.status(200).json({ message: 'Hello, world!' });
}
- Use Cases: Authentication, CRUD operations.
- Benefits: No need for an external server.
Client-side Fetching
Use fetch
or libraries like SWR for client-side data fetching.
import useSWR from 'swr';
const fetcher = (url) => fetch(url).then((res) => res.json());
function Component() {
const { data, error } = useSWR('/api/data', fetcher);
if (error) return <div>Failed to load</div>;
if (!data) return <div>Loading...</div>;
return <div>Data: {data.message}</div>;
}
- Use Cases: User interactions, live updates.
- Benefits: Dynamic and interactive.
- Use Cases: User interactions, live updates.
- Benefits: Dynamic and interactive.
3. Data Storage Options
External Databases
Integrate databases like PostgreSQL, MongoDB, or MySQL for robust data storage.
- Best Practice: Use environment variables to store database credentials.
Headless CMS
Connect to a headless CMS (e.g., Strapi, Contentful) to manage content efficiently.
- Benefits: User-friendly, scalable.
Local JSON Files
Suitable for small-scale apps or prototyping.
- Drawbacks: Limited scalability.
4. Client-Side Storage Mechanisms
LocalStorage
Store non-sensitive data persistently.
Session Storage
Temporary storage cleared after the session ends.
Cookies
Ideal for storing small amounts of sensitive data with proper security flags.
5. State Management in Next.js
React Context API
Lightweight solution for managing global state.
Redux
Use Redux for complex state management needs.
Zustand
A modern, minimalistic alternative to Redux.
6. Caching Strategies
Incremental Static Regeneration (ISR)
Update static pages without rebuilding the entire site.
Client-Side Caching with SWR
Leverage caching for faster client-side performance.
Server-Side Caching
Implement server-side caching to reduce database load.
7. Security Considerations
- Avoid storing sensitive data client-side.
- Validate and sanitize all user inputs.
- Implement proper authentication and authorization mechanisms.
8. Performance Optimization
- Use lazy loading to optimize initial page load.
- Minimize data fetching by combining requests.
- Employ server-side caching for better scalability.
9. Common Pitfalls and Solutions
- Hydration Mismatches: Ensure consistent server and client data.
- Error Handling: Gracefully handle API failures with fallbacks.
- Over-fetching Data: Optimize APIs to fetch only necessary data.
10. Conclusion
Data storage and management in Next.js offer a variety of tools and strategies to suit any application’s needs. By understanding and implementing the right methods, you can build efficient, scalable, and secure web applications with ease. Start experimenting today with the latest version of Next.js, and elevate your development experience!