How to implement Fonts in Next.js?
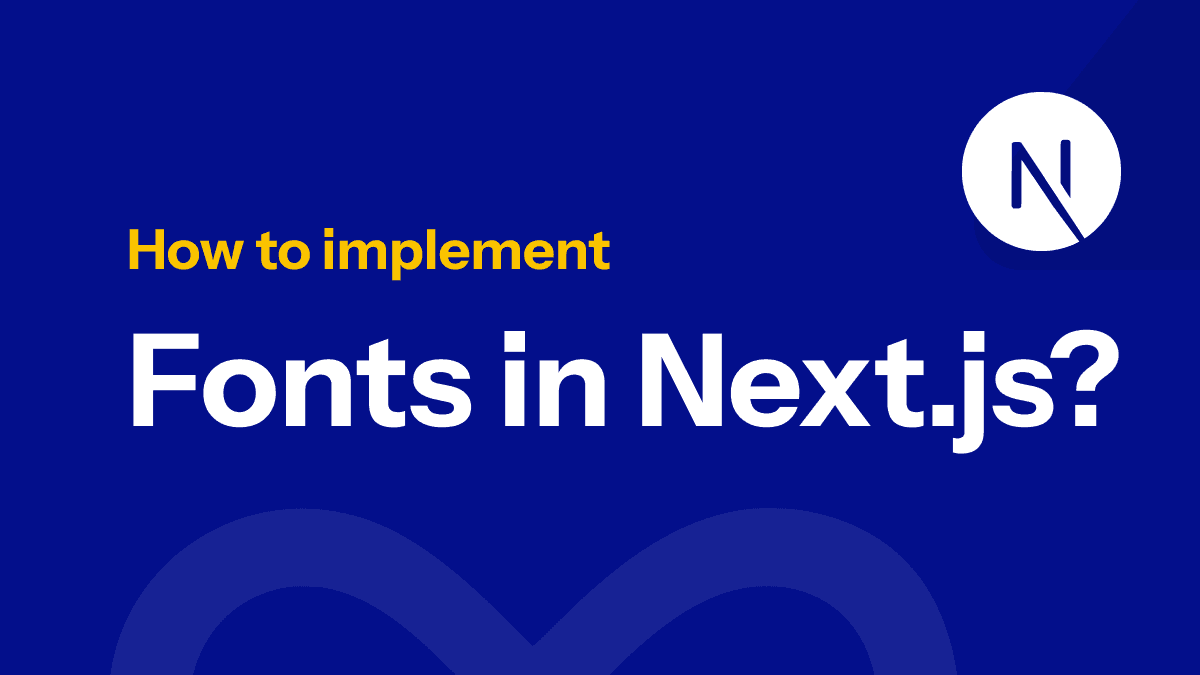
Typography plays a vital role in web design, making it crucial to use fonts effectively in your web application. In Next.js, implementing fonts is straightforward, thanks to features like the next/font
module, which simplifies font optimization and improves performance.
This guide will walk you through all the ways to implement fonts into a Next.js application, covering Google Fonts, custom fonts, and local fonts. We'll include practical examples, step-by-step instructions, and best practices.
Why Fonts Matter in Web Development
Fonts impact readability, branding, and overall user experience. Proper font implementation in Next.js ensures:
- Enhanced Performance: Optimized fonts improve load times.
- Privacy: Self-hosting fonts reduce third-party dependencies.
- Accessibility: Proper typography makes content easier to read for all users.
Next.js offers multiple ways to integrate fonts while prioritizing performance and user experience.
1. Using Google Fonts in Next.js
Using next/font/google
Font names in next/font/google
use PascalCase with underscores replacing spaces (e.g., 'Open Sans' becomes 'Open_Sans'). This naming convention ensures consistency when importing fonts. The next/font/google
module is the most optimized way to include Google Fonts in a Next.js application. It automatically self-hosts fonts, eliminates layout shifts, and improves privacy.
Step-by-Step Implementation
Font names in next/font/google
follow PascalCase, where spaces are replaced with underscores. For example, 'Open Sans' becomes 'Open_Sans'. This naming convention ensures consistency and compatibility.
- Import the Font: Import the desired font from
next/font/google
.
import { Open_Sans } from 'next/font/google';
const openSans = Open_Sans({
subsets: ['latin'], // Specify subsets for optimization
weights: ['400', '700'], // Optional: Define weights
styles: ['normal', 'italic'], // Optional: Define styles
});
Apply the Font Globally: In your app/layout.js
or app/layout.tsx
, apply the font to the <html>
or <body>
tag.
export default function RootLayout({ children }) {
return (
<html lang="en" className={openSans.className}>
<body>{children}</body>
</html>
);
}
Optional: Use CSS Variables: For selective font usage, define a CSS variable.
const openSans = Open_Sans({
subsets: ['latin'],
variable: '--font-open-sans',
});
Then, apply the font in your CSS:
body {
font-family: var(--font-open-sans), sans-serif;
}
Using Traditional <link>
Tag
Alternatively, you can include Google Fonts via a <link>
tag in _document.js
.
Step-by-Step Implementation
- Edit
_document.js
: Add the Google Fonts link inside the<Head>
tag:
import { Html, Head, Main, NextScript } from 'next/document';
export default function Document() {
return (
<Html lang="en">
<Head>
<link
href="https://fonts.googleapis.com/css2?family=Open+Sans:wght@400;700&display=swap"
rel="stylesheet"
/>
</Head>
<body>
<Main />
<NextScript />
</body>
</Html>
);
}
Apply the Font in CSS: Use the imported font in your global CSS file:
body {
font-family: 'Open Sans', sans-serif;
}
Pros and Cons
Method | Pros | Cons |
---|---|---|
next/font/google | Self-hosted, optimized, reduces FOUT | Slightly more setup |
<link> Tag | Simple to implement | External requests, possible layout shifts |
2. Adding Custom Fonts
Using Local Font Files
To include a custom font not available on Google Fonts, use the next/font/local
module.
Step-by-Step Implementation
- Organize Font Files: Place your font files (e.g.,
.woff2
,.woff
) in thepublic/fonts
directory. - Import the Font: Use
next/font/local
to define the font.
import localFont from 'next/font/local';
const customFont = localFont({
src: [
{ path: '/fonts/MyCustomFont-Regular.woff2', weight: '400', style: 'normal' },
{ path: '/fonts/MyCustomFont-Bold.woff2', weight: '700', style: 'normal' },
],
variable: '--font-custom',
});
3. Apply the Font: Set the font globally in layout.js
or via CSS variables.
export default function RootLayout({ children }) {
return (
<html lang="en" className={customFont.variable}>
<body>{children}</body>
</html>
);
}
body {
font-family: var(--font-custom), sans-serif;
}
3. Best Practices for Font Implementation
- Choose the Right Fonts:
- Use fonts that align with your brand and improve readability.
- Limit the number of font families to optimize performance.
- Optimize for Performance:
- Use
next/font
for self-hosting and reduced network requests. - Subset fonts to include only the characters you need.
- Use
- Ensure Accessibility:
- Use readable font sizes and contrast ratios.
- Test your typography across devices and browsers.
4. Common Pitfalls and How to Avoid Them
- Flash of Unstyled Text (FOUT):
- Use
next/font
to preload fonts and prevent layout shifts.
- Use
- Large Font Files:
- Subset fonts and compress font files for faster load times.
- Incorrect Font Paths:
- Ensure font files are placed correctly in the
public
directory.
- Ensure font files are placed correctly in the
- Overusing Fonts:
- Stick to a limited number of weights and styles to reduce load times.
Conclusion
Implementing fonts in Next.js is both flexible and efficient. Whether you’re using Google Fonts or custom fonts, Next.js provides tools to optimize performance and improve user experience. By following this guide, you can ensure your typography is both beautiful and functional.
Start enhancing your Next.js project with well-implemented fonts today!