How to Use Context API in Next.js 14: A Complete Guide

Simplifying State Management in Next.js Applications
Highlights:
- Learn how to use Context API in Next.js 14
- Simplify state management with
useContext
andcreateContext
- Explore practical examples using server and client components
State management is crucial when developing scalable applications. In Next.js 14, using the Context API for managing state simplifies data sharing across components. This guide explains how to set up and use the Context API in your Next.js application effectively.
Why Use the Context API in Next.js?
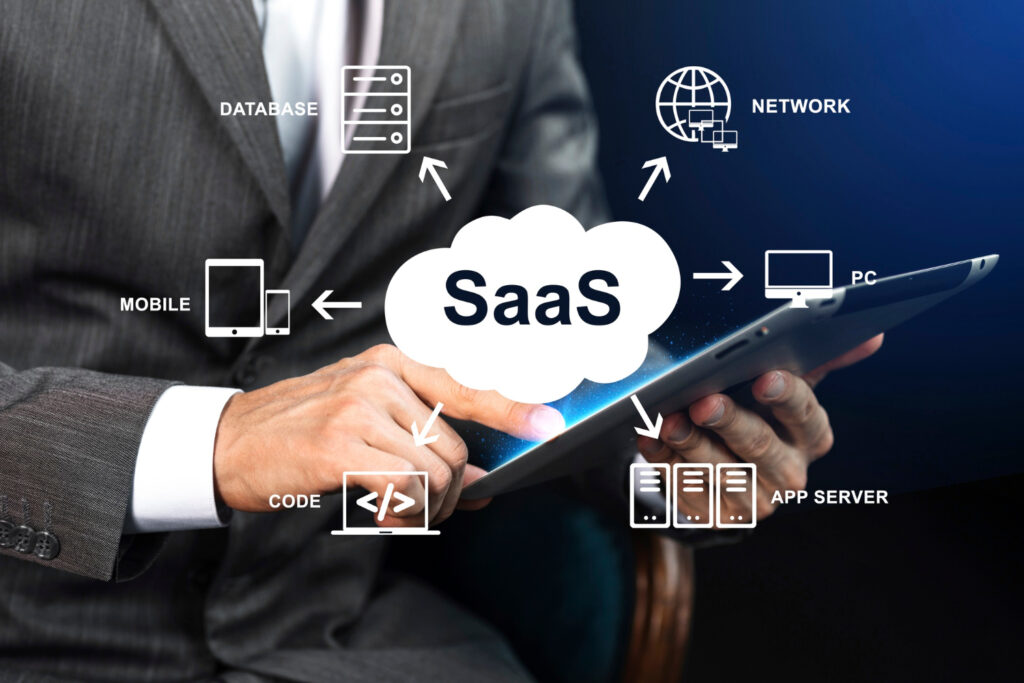
Next.js offers powerful tools for both server and client-side development. However, managing state across deeply nested components can be challenging. With Next.js Context, you can:
- Avoid "prop drilling" by passing data directly to components.
- Share global state using
createContext
anduseContext
hooks. - Simplify state management in both server components and client components.
For example, instead of passing a user object down multiple layers of components, you can use a Next.js Provider to make it accessible across the entire app.
Setting Up Context API in Next.js
Step 1: Create a Context
Start by creating a context using React’s createContext
function. In your Next.js application, define it as a separate module:
import { createContext, useContext, useState } from 'react';
export const UserContext = createContext(null);
export function UserProvider({ children }) {
const [user, setUser] = useState(null);
return (
<UserContext.Provider value={{ user, setUser }}>
{children}
</UserContext.Provider>
);
}
In this code:
createContext
: Creates a context object.UserProvider
: Wraps components and provides access to theuser
state.
Step 2: Use Context in Components
To access context values, use the useContext
hook. Here’s an example of consuming the UserContext
:
import { useContext } from 'react';
import { UserContext } from '../context/UserContext';
export default function UserProfile() {
const { user, setUser } = useContext(UserContext);
if (!user) {
throw new Error("No user data available");
}
return (
<div>
<h1>Welcome, {user.name}!</h1>
<button onClick={() => setUser(null)}>Logout</button>
</div>
);
}
Step 3: Wrap Your Application
Wrap your app with the UserProvider
in pages/_app.js
:
import { UserProvider } from '../context/UserContext';
export default function App({ Component, pageProps }) {
return (
);
}
his makes the user state available throughout the app.
Using Context API with Server Components
In Next.js 14, you can also pass context values to server components. For example, to pre-render a user profile on the server:
import { UserContext } from '../context/UserContext';
export default async function UserServerComponent() {
const user = await fetchUserData();
return (
Welcome, {user.name}!
);
}
async function fetchUserData() {
return { name: "John Doe", email: "john@example.com" };
}
This approach ensures SEO-friendly rendering while passing context data to server components.
Advanced Tips and Best Practices
Error Handling in Context
When consuming context values, always handle cases where the context might not be available:
const context = useContext(UserContext);
if (!context) {
throw new Error("UserContext must be used within a UserProvider");
}
Using Context with useState
Leverage useState
from React to manage local state within your context:
export const ThemeContext = createContext();
export function ThemeProvider({ children }) {
const [theme, setTheme] = useState('light');
return (
<ThemeContext.Provider value={{ theme, setTheme }}>
{children}
</ThemeContext.Provider>
);
}
Combining Context with Other Hooks
Combine useContext
with useEffect
to fetch and update data dynamically:
import { useEffect, useContext } from 'react';
import { UserContext } from '../context/UserContext';
export default function FetchUser() {
const { setUser } = useContext(UserContext);
useEffect(() => {
async function fetchData() {
const data = await fetch('/api/user').then((res) => res.json());
setUser(data);
}
fetchData();
}, [setUser]);
return <div>Loading user data...</div>;
}
Key Benefits of Context API in Next.js
- Effortless State Sharing: No need for complex state management libraries.
- Server and Client Compatibility: Works seamlessly with server-side rendering (SSR) and client components.
- Improved Code Readability: Cleaner structure without excessive props.
Conclusion
Using the Context API in Next.js 14 is a powerful way to manage global state. By combining createContext
, useContext
, and Next.js Provider, you can streamline state management for server and client components alike.
Whether you're building SEO-friendly applications with server-side rendering or dynamic client-side apps, the Context API offers a lightweight yet robust solution.
Explore more on Next.js state management in the official Next.js documentation and start simplifying your development workflow today!
Looking for a Next.js Developer? Hire now!